R.swift: Type-safe references to your images, storyboards and more
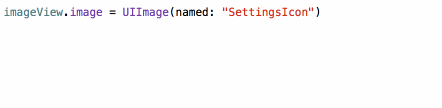
Every developer I know who switched from Objective-C to Swift loves the power of Swift. It has more compile time checks, it’s easier to write explicit state and there are quite nice functional patterns that you can use. Of course there is also a lot that can still be improved when using Swift, for example how UIKit still uses strings to identify resources.
While getting used to the compile time checks, we started to get a bit annoyed by errors due to mistyping image names, segue- and cell identifiers and only discovering such mistakes at runtime. And although images just don’t show up when you make a mistake, mistyping segue- and cell identifiers can easily crash your app. It’s unpleasant when just coding, and can become nerve-wracking when doing a large refactor.
We started thinking that there should be a better way than using string identifiers. So I decided to start developing R.swift to solve this problem. After about a year of active development, version 1.0 is released!
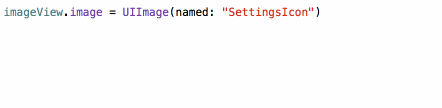
What R.swift does to make your life easier
R.swift gives you type-safe, compile time checked references to segues, images and more. It does this by checking, at compile time, whether the identifiers exist, and shows you compile errors if you made a typo or renamed something. On top of all that it even autocompletes images and other identifiers in Xcode!
For example, if you currently would write this:
let icon = UIImage(named: "settings-icon")
let font = UIFont(name: "San Francisco", size: 42)
let viewController = CustomViewController(nibName: "CustomView", bundle: nil)
With R.swift that would become:
let icon = R.image.settingsIcon()
let font = R.font.sanFrancisco(size: 42)
let viewController = CustomViewController(nib: R.nib.customView)
Many other things like cells, segues, storyboards and files are also supported. If you’re interested in how that would look, please take a look at the R.swift documentation.
So if you now decide to rename “settings-icon” to something else, the compiler will warn you about it:

We started using R.swift in all of our iOS projects and have seen that it saved us quite a few mistakes where the wrong typecast was used or when someone decided to rename an identifier. Give it a try and feel free to leave your feedback as a Github issue!
Mathijs explains R.swift 1.0:
Check out our Engineering Blog for more in depth articles on pragmatic code for happy users!